Type casting
- Ivaylo Fiziev
- Apr 24, 2023
- 2 min read
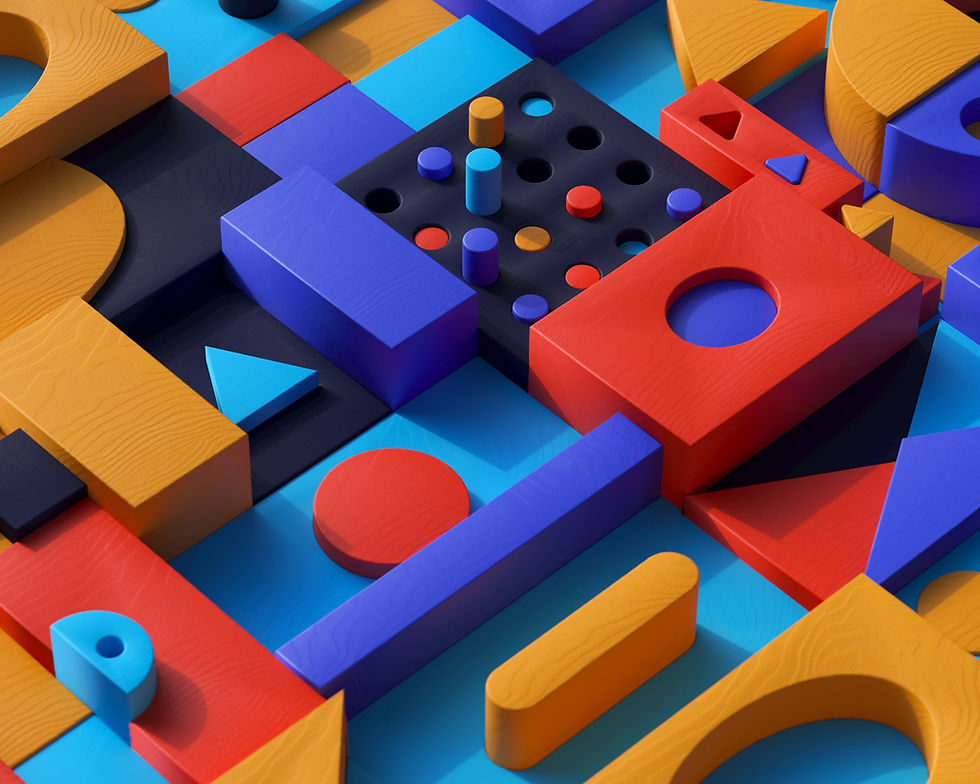
Types are good. They provide meaning to variables, arguments and functions by defining the set of operations that can be applied to them. The memory layout/footprint is also defined by the type. We are so used to the concept of types that usually we take them for granted.
But have you ever heard of Type System? If not this is the entity that assigns types to language structures like: literals, variables, functions, expressions etc. It is a mandatory part of every language. SCL is no exception. For example:
Boolean literals (True/False) are of type Bool
Integer literals (10,20,30) are of type DInt. If they don't fit in, the type is LInt.
Hex/Oct literals (16#FF, 8#77) are of type UDInt. If they don't fit in, the type is ULInt.
Floating point literals (3.14) are of type LReal
String literals ('hello') are of type String
Variable type is provided in it's declaration (var:Bool)
Function type is provided in its definition (FUNCTION "FOO" : Bool)
Expression type is determined based on its operands. If operand types are not compatible then the type of the expression cannot be determined and the expression is considered malformed (True AND 3.14) . We use the language grammar as a basis to check type compatibility.
Every now and then when we assign values to variables and function arguments we need to check if the type of the variable/argument matches the type of the expression used to provide the value.
Imagine we have a statement like this:
In this case we need to make sure that the type of the variable (left) matches the type of the expression (right). So what we do is ask the type system to determine the type of the variable and the expression and then compare them. If they do match we can go and do the assignment. If not we raise an error.
Now this is all fine but this makes the language way too strict.
Imagine if the variable is of integral type (DInt for example). In this case we will never be able to do the assignment this way. The user will have to change the expression type somehow. One way is to use a conversion function like this:
Actually this is the classic approach in SCL. You have a ton of conversion functions to handle each and every use case. This is also known as explicit type casting. e.g. the user should explicitly do something for the conversion to take place.
In out implementation of SCL we support the explicit way of doing type casts but also we allow for doing implicit casts. They only work on arithmetic expressions like the one above. Implicit means that we try to automatically do the cast based on the variable type.
Internally we do something like this:
Eventually if the cast is possible it will just be done. Otherwise an error will be raised.
To calculate expressions we also rely on the type system. In this case we determine the expression type first and then do something like this:
#out := var_type.cast(expr_type.and(expr_type.cast(#in.%X0), expr_type.cast(#in.%X1)));
Nice!
Comments