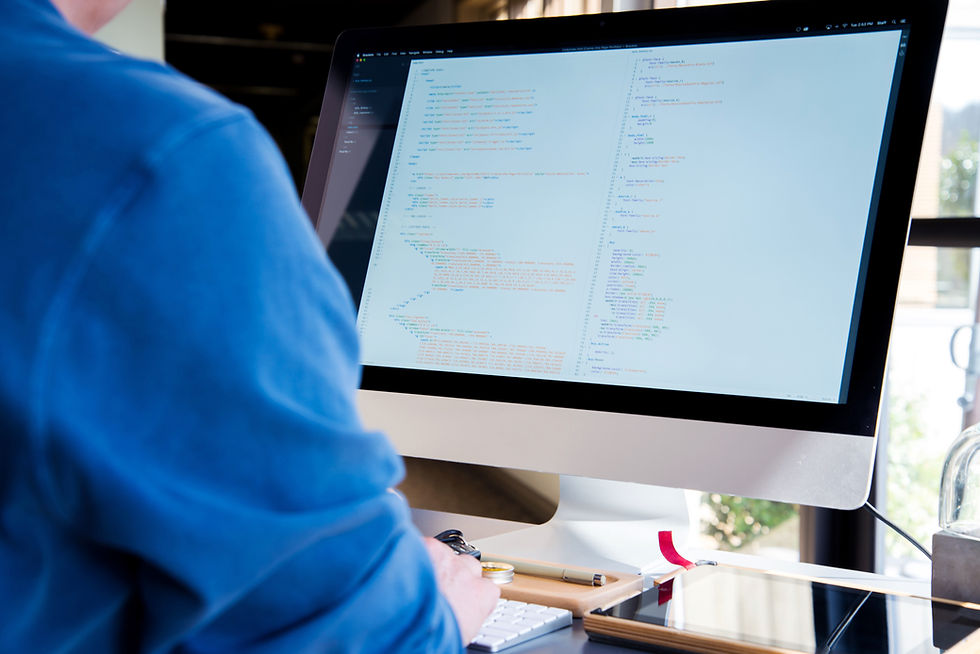
Statements that allow you to direct the execution of the program into alternative sequences of statements are known as control statements. In every programing language these are the primitives that make your code react to changes in the environment. Overall these are the conditional statements, unconditional jumps and the repetetive statments (loops).
The conditional statements are probably the first that come in handy when learning a new language. In SCL these are:
1. IF statement - enables you to drive the program execution into one of two alternative branches based on a boolean condition. The condition is provided in the form of a logical expression (logical expressions always evaluate to true or false).
Example:
; // do something
ELSIF #in = 20 THEN
; // do something else
ELSE
; // report error
END_IF;
Note: ELSIF/ELSE statements are optional. You can have any number of ELSIF statements but only a single ELSE statement. The first sequence of statements whose logical expression evaluates to true is executed. The rest are not executed.
2. CASE statement - enables you to drive the program execution into one of n alternative branches based on the value of an integral (numeric) expression.
Example:
CASE #in + 1 OF
1: ; // do something
2: ; // do something else
3,4,5: ; // do other thing
6..10: ; // do nothing
ELSE
; // report error
END_CASE;
Note: ELSE statement is optional. The first sequence of statements whose value maches the value of the expression is executed. The rest are not executed.
Repetetive statements allow a sequence of statements to be executed multiple times. In SCL these are:
1. FOR statement - executes the statements inside as long as the loop's control variable is within a specified range of values. The control variable must be of integral type. The start /end values must be of the same type as the control variable.
Example:
FOR #indx := 1 TO 20 BY 2 DO
; // do something
END_FOR;
Note: Increment (BY 2) is optional. If skipped the increment defaults to 1. The control variable will be automatically incremented.
2. WHILE statement - executes the statements inside as long as the loop's logical condition evaluates to true.
Example:
WHILE #in < 10 DO
; // do something
END_WHILE;
Note: The logical expression of the loop is evaluated before each execution. The counter should be incremented manually. If you miss it the loop will never end.
3. REPEAT statement - executes the statements inside until the loop's logical condition evaluates to true. Supported since (v2408)
Example:
REPEAT
; // do something
UNTIL #in > 10
END_REPEAT;
Note: The logical expression of the loop is evaluated after each execution. The counter should be incremented manually. If you miss it the loop will never end.
Unconditional jumps are used to change the program execution path immediately. As the name suggests no conditions are involved. The jump is direct. In SCL these are:
CONTINUE statement - terminates the execution of the current iteration of a loop statement. It immediately does so. If the control condition of the loop is not satisfied the body of the loop will execute again. Otherwise the loop ends. In case of nested loops the statement affects the innermost loop.
EXIT statement - terminates the loop at any point without checking the loop's control condition. In case of nested loops the statement affects the innermost loop.
RETURN statement - terminates the currently active block (FB/FC) and returns control to the calling block or Process Simulate. Supported since v2402.
GOTO statement - not supported (v2402).
What's supported in the script? More and more I get this question from customers.
I hope this clears things up a bit. We build SCL incrementally based on customer feedback. So the more feedback we get the more syntax will be covered.
Enjoy!
Comments