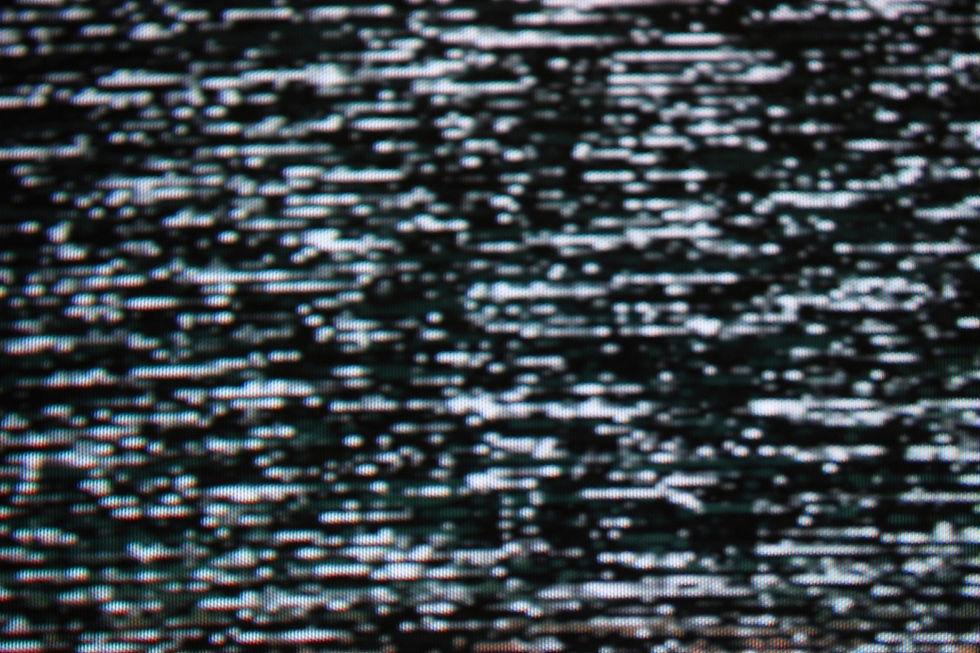
A noise generator by definition produces a random signal. In the software world this usually means generating pseudo-random numbers. One of the oldest algorithms used to do this is the linear congruential generator (LCG). It relies on a single equation that is easy to understand and use. Wikipedia has this well explained: https://en.wikipedia.org/wiki/Linear_congruential_generator
In the context of the noise generator we will use this algorithm to generate the output.
The LCG is implemented as a SCL function:
FUNCTION "LCG" : INT
VAR_INPUT
a:INT; // 0 < a < m
c:INT; // 0 <= c < m
m:INT; // 0 < m
seed:INT; // 0 <= seed < m
END_VAR
BEGIN
#LCG := (#a * #seed + #c) mod #m;
END_FUNCTION
As always this FC should be put in the SCL vault - LCG.scl
In our main block we'll call this function periodically, preserving the result of it between script executions. The result is then used as an input to the next call.
FUNCTION_BLOCK "MAIN"
VERSION:1.0
VAR_IN_OUT
seed : INT := 0; // start seed
END_VAR
BEGIN
#seed := "LCG"(m:=9, a:=2, c:=0, seed:=#seed); // next seed
END_FUNCTION_BLOCK
When you wire the 'seed' to a signal you can observe the output in simulation panel. The initial value of the signal provides the staring seed.
Try it. It works really well despite the simplicity.
Impressive!
Comentários