Bit Indexers
- Ivaylo Fiziev
- Mar 24, 2023
- 2 min read
Updated: Apr 18, 2024

I am sure you have used bitwise operations in your practice but have you heard of bit indexers? Personally I didn't know this concept at all before working on SCL. At first they seem a little odd but once you start using them they really come in handy. So what are they?
Bit indexers allow for accessing individual bits of an integral variable. Each bit is treated like a BOOL variable. This save you the effort of putting bit masks in your code. The bit masks are automatically created for you instead. Eventually this makes the code cleaner and more easy to understand.
Here are some examples:
Let's suppose you have a variable of type DWORD (flags) and you need to read the least significant bit in it. In this case you can simply type - #flags.%X0 - and this will return either TRUE or FALSE depending on the state of the first bit. Now you can use this expression in any context you like.
Imagine you have to detect a rising edge on the first bit. Then you can use the rising edge trigger that we already know about and call it like this:
#trigger(CLK:=#flags.%X0); // bit indexer
#trigger(CLK:=#flags AND 16#1); // bit mask
When setting the value of individual bits the statements will look like this:
#flags.%X0 := TRUE; // bit indexer
Q:Can I access more than one bit at the same time?
A:Yes. You have Byte, Word, Dword indexers as well. However in Process Simulate (as of version 2304) Dword indexers are still not implemented.
Using the example from above we can show the usage of these:
Reading from the least significant byte:
#flags.%B0 // byte indexer
#flags AND 16#FF // bit mask
Writing to the least significant byte:
#flags.%B0 := 5; // byte indexer
#flags := (#flags AND 16#FFFFFF00) OR 5; // bit mask
Reading from the least significant word:
#flags.%W0 // word indexer
#flags AND 16#FFFF // bit mask
Writing to the least significant word:
#flags.%W0 := 5; // byte indexer
#flags := (#flags AND 16#FFFF0000) OR 5; // bit mask
Q: What would happen if you provide an invalid index? #flags.%X121 ?
A: The result of this expression will always be FALSE. If you try to assign a value using an invalid index (#flags.%X121 := TRUE) the value won't be modified. Maybe we can think about raising an error in this case.
Q: What would happen if you try to use Bit/Byte/Word indexers on a variable of non- integral type?
A: You'll be presented with an error:
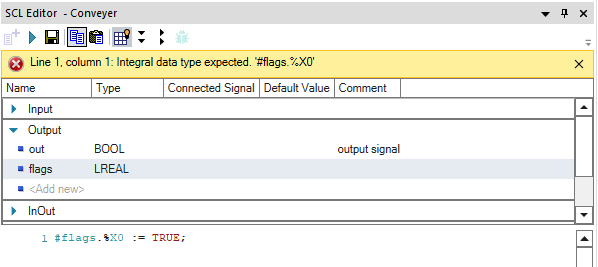
Congratulations! Now you have one more syntax weapon on your belt. I hope you'll find it useful.
Have a wonderful day!
Ivaylo
Update 1: In version 2408 Dword indexers (.%D0) are now implemented.
Comments