Behavior modeling with scripts
- Ivaylo Fiziev
- Apr 19, 2022
- 2 min read
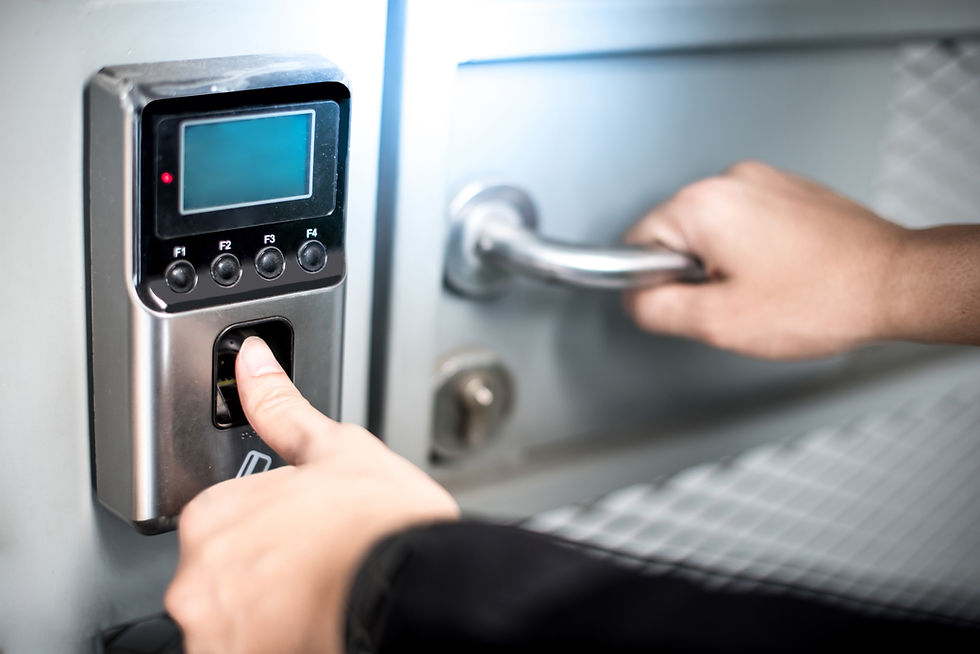
What is a script? A sequence of instructions (statements) executed one by one by the language interpreter. Each instruction gets you closer to achieving the goal of the script whatever that is. You put some effort designing the script so it covers every possible use case that you have. Once the script is ready you can use it as a building block when writing more complex scripts. Eventually you'll have a number of scripts that you can reuse in several different situations. Now this is where the behavior modeling becomes easy.
The behavior model can be as simple as inverting the input of your script.
FUNCTION "Invert" : BOOL
VAR_INPUT
in: BOOL;
END_VAR
BEGIN
END_FUNCTION
Now you can invert a Boolean value wherever needed. It is a simple model but it is all in your hands. You define the behavior that you need. You don't need some fancy stuff to do this. It is only a text file. You can change your model when needed. Fix bugs or redesign it from scratch. Isn't this lovely?
SCL has some built in FBs/FCs that will help you do yours with ease. To mention a few: timers, counters, edge triggers - common functionality that is widely used.
Imagine that you need to invert the input when a clock signal changes from 0 to 1.
This time we should use a FB. Why? It is because we need a static variable.
FUNCTION_BLOCK "Invert"
VAR_INPUT
clk: BOOL;
in: BOOL;
END_VAR
VAR_OUTPUT
out: BOOL;
END_VAR
VAR
trigger: R_TRIG;
END_VAR
BEGIN
#trigger(CLK:=#clk); // detect clock change
if #trigger.Q then // check for clock change
END_FUNCTION_BLOCK
There you have it. Your own FB that uses a built-in FB.
How about detecting a transition from 1 to 0? No problem. Just use F_TRIG instead of R_TRIG. Simple change in the text but a big change in the logic.
I think you get the idea.
Modeling is easy with scripts!
Reusing scripts is a big thing!
Comentários