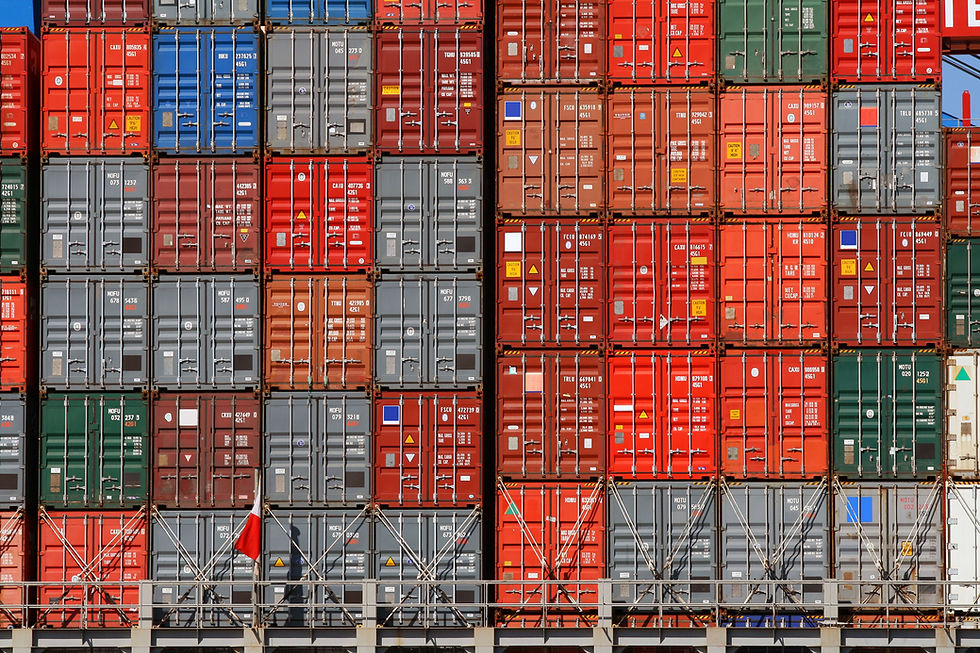
Now that we know what a variable is imagine a use case where you need a number of them. Maybe ten, twenty or more? What would you do? Declare each and every one of them? Of course not. You just create an array instead.
Arrays in SCL are sequential data structures that provide fast access to the elements inside. The lower bound and the size of the array are predefined and cannot be changed at runtime. All elements of the array share the same data type. e.g. arrays cannot be heterogeneous. Arrays can be multi-dimensional. In Process Simulate SCL supports up to three dimensions.
Arrays of arrays are not supported.
How do you declare an array?
Example:
VAR
arr : Array [1..10] of BOOL; // declare an array of 10 elements starting at index 1
mtx : Array [1..10,1..10] of BOOL; // declare a matrix of 10x10 elements starting at index 1 in each dimension
END_VAR
How do you use an array?
Example:
BEGIN
#arr[1] := True; // assign value - index is a decimal literal
#arr[#indx] := True; // assign value - index is an integral variable
#mtx[1,2] := True; // assign value - index is decimal literal
if #arr[1] <> 1 then ... // consume value - index is a decimal literal
if #arr[#indx] <> 1 then ... // consume value - an integral variable
if #mtx[1,2] <> 1 then ... // consume value - an integral variable
Any SCL data type can be used to create arrays. The default value (zero) for the data type will be used to provide initial values for the elements of the array.
Note: If the array is used in a temporary variable the array will be recreated on each execution of the script. Depending on the size of the array this might affect performance.
Comments